Internationalization and localization
Easystart’s default language is English, so all text will be in English by default. However, it also has a Spanish translation available since it’s the second most spoken language. Don’t worry if your preferred language is not available, we’ll show you how to add a new language to the system.
How to add a new language
Backend
To practice, let’s attempt to add the French language to the system. Firstly, we need to modify the settings.py
file and add the new language to the configuration by
updating the LANGUAGES
list.
LANGUAGES = [
("fr", "French"),
("es", "Spanish"),
("en-us", "English"),
]
To ensure the translation command detects an app’s translation files, you need to make sure that the app is included in the JS_INFO_DICT
dictionary.
While all apps are included by default, if you want to add a new module, you’ll need to add it yourself. You can find this variable in the settings.py
file and it has a specific structure.
JS_INFO_DICT = {
"packages": (
"apps.core",
"apps.accounts",
"apps.two_factor_auth",
"apps.management",
"apps.audit",
"apps.wallet",
)
}
Create a directory called fr/LC_MESSAGES
in the locale
folder of each application that doesn’t already have one.
apps
├── accounts
│ ├── locale
│ ├── ├── fr/LC_MESSAGES
After creating the fr/LC_MESSAGES
directory, we need to create the djangojs.po
file inside it.
This file is where we will insert the translation pair from English text to French text.
There are two ways to write the translation pair:
When the text is static and does not contain any variable attributes:
msgid "Welcome"
msgstr "Bienvenue"
When the text contains a value that may vary dynamically:
msgid "Max. size %(size)s MB"
msgstr "Taille maximale %(size)s Mb"
In this case, the size will be passed as an argument and inserted into the text.
Note
Please note that the text before the msgid
key represents the original text and the msgstr
represents the translated text.
If you want to learn more about translation in Django, you can visit https://docs.djangoproject.com/en/4.1/topics/i18n/translation/
Frontend
In the frontend, there are two global methods that can be used to get the translation of a key text (originally in English) based on the selected language.
These methods are $_
and $i_
. Let’s take a look at how they work.
To translate a text without a dynamic value, we use the $_
method:
<div>
<p>{{ $_("Welcome") }}</p>
</div>
// The expected result is
<div>
<p>Bienvenue</p>
</div>
To translate dynamic texts, both methods must be combined ($_
y $i_
):
<div>
<p>{{ $i_($_('Max. size %(size)s MB'), {size: 8 }, true) }}</p>
</div>
// The expected result is:
<div>
<p>Taille maximale 8 Mb</p>
</div>
Backend and Frontend Integration
You might wonder how the frontend can access the translation pairs defined in the backend. The answer is simple: the backend provides a file called
translation-[hash].js
with all the translation pairs on the first load. To make this happen, you need to execute the following commands:
docker compose exec applocal python manage.py compilemessages
docker compose exec applocal python manage.py generate_i18n_js static/js/translation
The first command compiles all the djangojs.po
files to generate a compiled file with the translation pairs. The second command creates the translation-[hash].js
file
and places it in the static/translation
directory. Afterwards, it is added to the base.html
template, allowing the frontend to access it at any time.
Note
Each time a translation pair is added or updated, both commands must be executed.
How to change the language
The default language for a user is registered in the UserProfile
model of the core
app, and it is passed from the backend as props.
This means that every time a user logs in, their preferred language is available, and the frontend performs the translation accordingly.
However, if the user is not logged in, the frontend detects the default language set by the browser.
If the browser language is not among the languages set in the application, the default language is used.
For a first-time user, the texts will appear in the default Easystart language (English).
To change this, the user must go to the Account Settings
section and modify the language preference under the Language
label.
English Dashboard
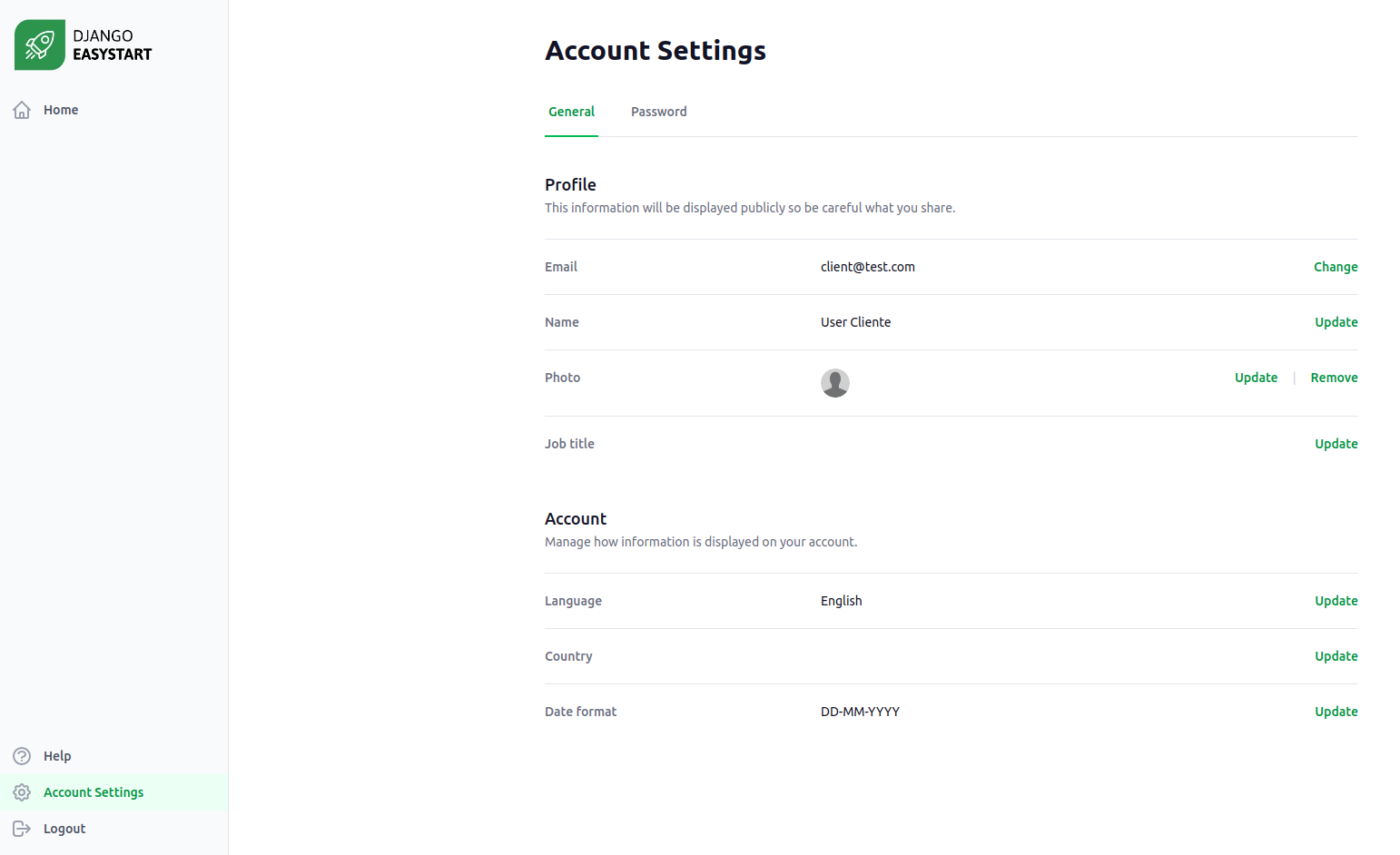
Spanish Dashboard
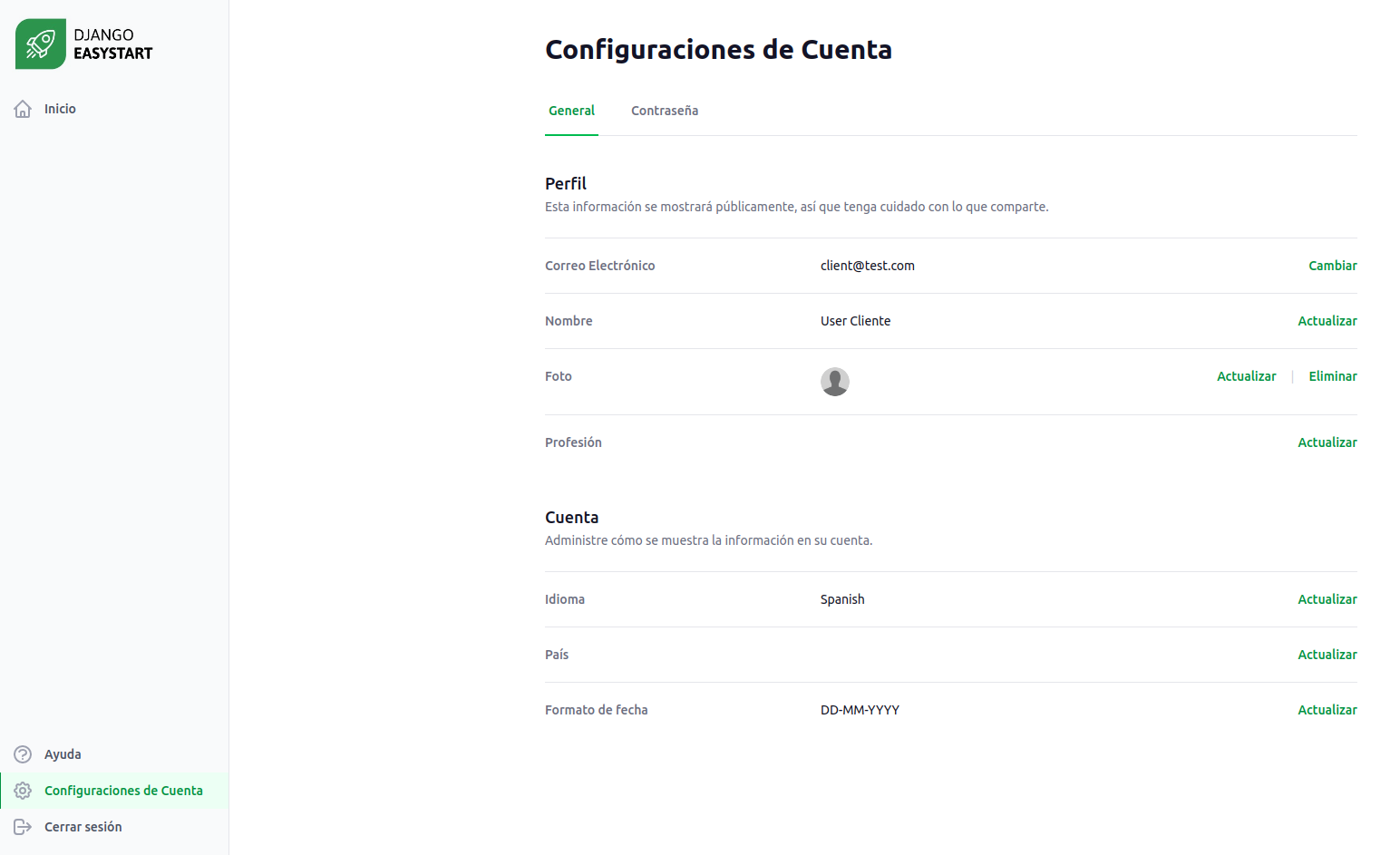